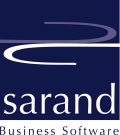 |
Perl Operators
|
|
Operators |
Unlike many languages, numeric and string values have different operators
that act upon them. The table of precedence follows at the end, but the
following paragraphs identify the common operators. |
Assignment operator |
= |
The assignment operator for numeric and string data. |
The assignment operator returns the variable that was assigned to,
so:
($a += 3) *= 2; # Is
equivalent to $a += 3; $a *= 2; |
The assignment operator can be combined with a range of other operators
to provide a shortcut, eg.
$a += $b; # Equivalent to
$a = $a + $b;
The following operators can be combined in this way:
**= += -= .=
*= /= %= x=
&= |= ^=
<<= >>= &&= ||= |
Quote operators |
Quotes act as operators in Perl, supporting interpretation of their
contents (interpolation) and pattern matching.
Although a set of customary quote styles is expected for certain operations,
it is possible to use any pair of delimiters (substituting for [] below): |
Customary
|
Generic
|
Meaning
|
Interpolates
|
''
|
q[]
|
Literal
|
no
|
""
|
qq[]
|
Literal
|
yes
|
``
|
qx[]
|
Command
|
yes
|
|
qw[]
|
Word list
|
no
|
//
|
m[]
|
Pattern match
|
yes
|
|
s[][]
|
Substitution
|
yes
|
|
tr[][]
|
Translation
|
no
|
For constructs that perform interpolation, the following escape sequences
are supported: |
\t |
tab |
\n |
newline |
\r |
return |
\f |
formfeed |
\v |
vertical tab |
\b |
backspace |
\a |
alarm (bell) |
\e |
esccape |
\c[ |
control char |
\l |
lowercase next char |
\u |
uppercase next char |
\Q |
quote regexp metacharacters till \E |
\L |
lowercase till \E |
\U |
uppercase till \E |
\E |
end case modification |
\0nn |
octal char |
\xhh |
hex char |
|
|
Where variables and other regular expressions form part of a pattern,
these are interpreted befor ethe pattern matching, thus allowing the pattern
to have variable portions. Other than this two stage interpolation,
Perl does not interpret embedded quotes as a further level of delimiter.
Thus:
$a = "Hello"; # $a becomes Hello
$b = "'$a'"; # $b becomes
'Hello'
$c = '"$a"'; # $c becomes
"$a" |
A string enclosed by backticks (`...` - grave accents) undergoes variable
substitution like a double quoted string, but is then interpreted as a
command, returning the output of that command. In a scalar context, a single
string consisting of all the output is returned. In a list context, a list
of values is returned, one for each line of output, $/
determining the line terminator. The command is executed each time the
expression is evaluated. The status value of the command is returned in
$?.
Unlike in the shell, no translation is done on the return data (newlines
remain newlines) and single quotes do not hide variable names in the command
from interpretation. To pass a $ through to the shell you need to hide
it with a backslash. The generic form of backticks is qx//. |
Symbolic unary operators |
! |
logical negation (also 'not') |
- |
arithmetic negation |
~ |
bitwise negation (1's complement) |
+ |
No effect, but useful to delimit certain expressions. |
\ |
Creates a reference to whatever follows it |
=~ |
binds an expression to a pattern match, that may be a search pattern,
substitution or translation. The return value indicates the operation's
success:
$success = ($data =~ $pattern);
The default for the data variable is '$_'. |
!~ |
As above, except the return value is negated |
* |
Muliplication |
/ |
Division |
% |
Modulus (remainder) |
x |
Repetition, performed on either a string or a list |
+ |
Sum of two numbers |
- |
Difference between two numbers |
. |
Concatenation of two strings |
<< |
Bitwise shift left on an integer target |
>> |
Bitwise shift right on an integer target |
< |
Less than (numerical) |
> |
Greater than |
<= |
Less than or equal |
>= |
Greater than or equal |
lt |
Less than (stringwise) |
gt |
Greater than |
le |
Less than or equal |
ge |
Greater than or equal |
== |
Equal (numerical) |
!= |
Not equal |
<=> |
Returns:-1 for less than, 0 for equal, 1 for greater than. |
eq |
Equal (stringwise) |
ne |
Not equal |
cmp |
As above for '<=>'. |
& |
Bitwise 'AND' |
| |
Bitwise 'OR' |
^ |
Bitwise 'XOR' |
Autoincrement |
The '++' operator increments its target either before its value is
returned (placed to the left) or after:
$a = 1;
$b = ++$a; # $a = 2, $b =
2
$c = $a++; # $a = 3, $b =
2, $c = 2
$d = --$a; # $a = 2, $d =
2
$e = $a--; # $a = 3, $e =
2 |
Autoincrement (but not autodecrement) also works with alphanumerics,
such that:
++($var = 'm7') is equivalent to 'm8'
++($var = 'kZ') is equivalent to 'lA' |
Logical operators |
&& |
Logical AND, returning the last expression evaluated. If the left operand
is false, the right is not evaluated. |
|| |
Logical OR, returning the last expression evaluated. If the left operand
is true, the right is not evaluated. |
and |
Equivalent to '&&' with lower precedence. |
or |
Equivalent to '||' with lower precedence. |
xor |
Logical XOR, both expressions evaluated, right expression returned. |
? |
Conditional operator:
CONDITION ? TRUE_RESULT : FALSE_RESULT; |
.. |
In a list context, returns an array of incremented values between the
two values specified (incluseive). |
.. |
In a scalar context, returns a boolean value, acting as a bistable
operatorr (something of a flip-flop). |
... |
In a scalar context, equivalent to '..' except in specific actions. |
, |
In a scalar context, the left argument is evaluated first, and then
the right. The result of the last evaluation (right hand side) is
returned. |
, |
In a list context, this is the argument separator. |
IO operators |
Filehandles are created with the open()
function. Either a filehandle (no prefix) or a variable can be specified,
where the variable holds the file handle:
$filUser = 'USER';
open($filUser); # Equivalent
to open(USER); |
Angle brackets around a file handle returns the next line from that
file, delimited by $/ (default to
\n). The delimiter is included, so the value is not false until end of
file, at which time an undefined value is returned. Usually the value must
be assigned to a variable, but if the input symbol is the only thing inside
the conditional of a while loop, the value is automatically assigned to
the variable $_. The following lines
are equivalent to each other:
while ($_ = <STDIN>) { print; }
# Also <$fil> where $fil holds the handle.
while (<STDIN>) { print; }
for (;<STDIN>;) { print; }
print while $_ = <STDIN>;
print while <STDIN>; |
If <FILEHANDLE> is used in a list context, a list of all the input
lines is returned, one line per list element:
@users = <USERS>; # Beware
the volume of data retrieved. |
File Test operators |
The '-X EXPR' file test performs the specified condition on the FileHandle
or FileName as listed below: |
-r |
File is readable by effective user/group |
-w |
File is writable by effective user/group |
-x |
File is executable by effective user/group |
-o |
File is owned by effective user/group |
-R |
File is readable by real user/group |
-W |
File is writable by effective user/group |
-X |
File is executable by real user/group |
-O |
File is owned by effective user/group |
-e |
File exists |
-z |
File has zero size |
-s |
File has non-zero size (returns size) |
-f |
File is a plain file |
-d |
File is a directory |
-l |
File is a symbolic link |
-p |
File is a named pipe (FIFO) |
-S |
File is a Socket |
-b |
File is a block special file |
-c |
File is a character special file |
-t |
Filehandle is opened to a tty |
-u |
File has setuid bit set |
-g |
File has setgid bit set |
-k |
File has sticky bit set |
-T |
File is a text file |
-B |
File is a binary file (opposite of -T) |
-M |
Age of file in days when script started |
-A |
Same for access time |
-C |
Same for inode change time |
|
|
Precedence |
The operators in Perl attract the following priority when it comes
to their evaluation (very similar to 'C'): |
-
left terms and list operators (leftward)
-
left ->
-
nonassoc ++ --
-
right **
-
right ! ~ \ and unary + and -
-
left =~ !~
-
left * / % x
-
left + - .
-
left << >>
-
nonassoc named unary operators
-
nonassoc < > <= >= lt gt le ge
-
nonassoc == != <=> eq ne cmp
-
left &
-
left | ^
-
left &&
-
left ||
-
nonassoc ..
-
right ?:
-
right = += -= *= etc.
-
left , =>
-
nonassoc list operators (rightward)
-
left not
-
left and
-
left or xor
|
|
|