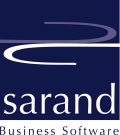 |
Perl Functions
|
|
A-B-C-D-E-F-G-H-I-J-K-L-M-N-O-P-Q-R-S-T-U-V-W-X-Y-Z
abs VALUE |
Returns the absolute value of the argument. |
accept NEWSOCKET,GENERICSOCKET |
Accepts an incoming socket connect as the accept system call
does. Returns the packed address if it succeeded, otherwise False. |
alarm SECONDS |
Arranges to have a SIGALRM delivered to this process after the specified
number of seconds have elapsed. Only one timer can be used at a time. Each
call disables the previous timer, and an argument of 0 may be supplied
to cancel the previous timer without starting a new one. The returned value
is the amount of time remaining on the previous timer. |
atan2 Y,X |
Returns the arctangent of Y/X in the range -PI to PI. |
bind SOCKET,NAME |
Binds a network address to a socket, just as the bind system
call does. Returns True on success, otherwise False. NAME
should be a packed address of the appropriate type for the socket. |
binmode FILEHANDLE |
Arranges for the file to be read or written in "binary" mode in operating
systems that distinguish between binary and text files. Files that are
not in binary mode have CR LF sequences translated to LF on input and LF
translated to CR LF on output. Binmode has no effect under Unix; in DOS,
it may be imperative. |
bless REF,PACKAGE |
This function tells the referenced object (passed as REF) that it is
now an object in PACKAGE (defaults to the the current package). It returns
the reference for convenience, since a bless() is often the last thing
in a constructor. |
caller EXPR
caller |
Returns the context of the current subroutine call. In a scalar context,
returns True if there is a caller, ie. when in a subroutine or eval()
or require(), otherwise False. In a list
context, returns:
($package,$filename,$line) = caller;
With EXPR, it returns some extra information that the debugger uses
to print a stack trace. The value of EXPR indicates how many call frames
to go back before the current one. |
chdir EXPR |
Changes the working directory to EXPR (defaults to home directory),
if possible. Returns True on success, otherwise False. See
die(). |
chmod LIST |
Changes the permissions of a list of files. The first element of the
list must be the numerical mode. Returns the number of files successfully
changed.
$cnt = chmod 0755, 'abc', 'xyz';
chmod 0755, @executables; |
chomp VARIABLE
chomp LIST
chomp |
This is a slightly safer version of chop.
It removes any line ending that corresponds to the current value of $/,
returning the number of characters removed. It's often used to remove the
newline (or other record delimiter) from the end of an input record. In
paragraph mode ($/ = ""), it removes all trailing newlines from the string.
while (<>) # Read from StdIn
{
chomp; #
Remove delimiter (newline)
...
}
Any valid lvalue can be chomped:
chomp($answer = <STDIN>);
If you chomp a list, each element is chomped, and the total number
of characters removed is returned. |
chop VARIABLE
chop LIST
chop |
Chops off the last character of a string (defaults to $_)
and returns the character chopped. It's used primarily to remove the newline
from the end of an input record, but is much more efficient than s/\n//
because it neither scans nor copies the string. Examples as per chomp
above. |
chown LIST |
Changes the owner (and group) of a list of files. The first two elements
of the list must be the NUMERICAL uid and gid, in that order. Returns the
number of files successfully changed.
$i = chown $uid, $gid, 'abc', 'xyz';
chown $uid, $gid, @filenames; |
chr NUMBER |
Returns the character represented by that NUMBER in the character set.
For example:
$sA = chr(65); # "A"
in ASCII. |
chroot FILENAME |
Does the same as the system call of that name. FILENAME defaults to
$_. |
close FILEHANDLE |
Closes the file or pipe associated with the file handle, returning
True
only if stdio successfully flushes buffers and closes the system
file descriptor. Open explicitly closes a FileHandle
before attempting to open the file, but this does not reset the line counter
($.) in the way that an explicit
close does. Also, closing a pipe will wait for the process executing on
the pipe to complete, in case you want to look at the output of the pipe
afterwards, and puts the status value of the command into $?.
Example:
open(OUTPUT, '|sort >tmp'); # pipe to sort
...
# write data to sort via 'OUTPUT'
close OUTPUT;
# wait for sort to finish
open(INPUT, 'tmp');
# get sort's results |
closedir DIRHANDLE |
Closes a directory opened by opendir. |
connect SOCKET,NAME |
Attempts to connect to a remote socket, just as the connect system
call does. Returns True on success, otherwise False. |
cos EXPR |
Returns the cosine of EXPR expressed in radians (defaults to $_). |
crypt PLAINTEXT,SALT |
Encrypts a string exactly like the crypt function in the C library.
Useful for checking the password file , amongst other things. An example
that checks the password for the current session:
$pwd = (getpwuid($<))[1];
# Retrieve encrypted password
$salt = substr($pwd, 0, 2); # Encryption
key
...
# Retrieve password from user etc.
if (crypt($word, $salt) ne $pwd)
{ die "Password does
not match.\n"; } |
dbmclose ASSOC_ARRAY |
Breaks the binding between a DBM file and an associative array. Superseded
by untie. |
dbmopen ASSOC,DBNAME,MODE |
This binds a dbm or ndbm file to an associative array
where ASSOC is the name of the associative array and not a filehandle.
DBNAME is the name of the database (without the .dir or .pag
extension). If the database does not exist, it is created with protection
specified by MODE (as modified by the umask). If
the system has neither DBM nor ndbm, calling dbmopen() produces a fatal
error. Superseded by tie. |
defined EXPR |
Returns a boolean value saying whether the expression represents a
defined value. When used on a hash array element, it tells you whether
the value is defined, not whether the key exists in the hash. |
delete EXPR |
Deletes the specified value from its hash array, returning the deleted
value, or undefined if nothing was deleted.
delete $ARRAY{$key}; |
die LIST |
Outside of an eval, prints the contents of LIST
to STDERR and exits with the current value of $!
(errno). If $! is 0, exits with the value of ($? >> 8) (`command` status).
If ($? >> 8) is 0, exits with 255. Inside an eval,
the error message is stuffed into $@
and the eval is terminated with the undefined value. If the value of EXPR
does not end in a newline, the current script line number and input line
number (if any) are also printed, and a newline is supplied. |
do BLOCK |
Returns the value of the last command in the sequence of commands indicated
by BLOCK. When modified by a loop modifier, executes the BLOCK once before
testing the loop condition. |
do EXPR |
Uses the value of EXPR as a filename and executes the contents of the
file as a Perl script. Its primary use is to include subroutines from a
Perl subroutine library:
do 'stat.pl';
This is just like :
eval `cat stat.pl`;
except that it's more efficient, more concise, keeps track of the current
filename for error messages, and searches all the -I libraries if the file
isn't in the current directory. It's the same, however, in that it does
reparse the file every time it is called.
The inclusion of library modules is better done with the use
and require operators. |
dump LABEL |
This causes an immediate core dump. |
each ASSOC_ARRAY |
Returns a 2 element array consisting of the key and value for the next
entry in an associative array. Entries are returned in an apparently random
order. When the array is entirely read, a null array is returned and the
next call to each() after that will start iterating again. The iterator
can be reset only by reading all the elements from the array. Elements
should not be added to the array while it is being iterated.
while (($key,$value) = each %ENV)
{ print "$key = $value\n"; }
See also keys and values. |
eof FILEHANDLE
eof |
Returns 1 if the next read on FILEHANDLE will return end of file, or
if FILEHANDLE is not open.
# Insert dashes just before last line of last
file:
while (<>)
{
if (eof()) { print "--------------\n";
}
print;
} |
eval EXPR|BLOCK |
EXPR (defaults to $_) is parsed
and executed as if it were a little Perl program. It is executed in the
context of the current Perl program, so that any variable settings, subroutine
or format definitions remain afterwards. The value returned is the value
of the last expression evaluated, or a return statement may be used, just
as with subroutines. |
If there is a syntax error or runtime error, or a die() statement is
executed, an undefined value is returned by eval, $@
is set to the error message. If there was no error, $@ is guaranteed to
be a null string. The final semicolon, may be omitted from the expression. |
exec LIST |
The exec() function executes a system command without returning. The
system
function should be used if a return is required |
exists EXPR |
Returns True if the specified key exists in its hash array,
even if the corresponding value is undefined. |
exit EXPR |
Evaluates EXPR and exits immediately with that value. If EXPR is omitted,
exits with 0 status. |
exp EXPR |
Returns 'e' (the natural logarithm base) to the power of EXPR (defaults
to
$_). |
fcntl FILEHANDLE,FUNCTION,SCALAR |
Implements the fcntl function. Argument processing and value
return works just like ioctl below. Produces a fatal
error if used in a context that doesn't implement fcntl.
use Fcntl;
fcntl($filehandle, F_GETLK, $packed_return_buffer); |
fileno FILEHANDLE |
Returns the file descriptor for a filehandle. This is useful for constructing
bitmaps for select. If FILEHANDLE is an expression,
the value is taken as the name of the filehandle. |
flock FILEHANDLE,OPERATION |
Applies a lock to the specified file, returning True if successful:
$LOCK_SH = 1;
$LOCK_EX = 2;
$LOCK_NB = 4;
$LOCK_UN = 8;
flock($handle,$LOCK_EX); |
fork |
Performs a fork system call, returning the child pid
to the parent process and 0 to the child process, or undef if unsuccessful. |
formline PICTURE, LIST |
Formats a list of values according to the contents of PICTURE, placing
the output into the format output accumulator, $^
A. When a write is done, the contents of $^ A
are written. |
getc FILEHANDLE
getc |
Returns the next character from the input file attached to FILEHANDLE
(defaults to STDIN), or a null string at end of file. |
getlogin |
Returns the current login from /etc/utmp, if any. If null, use
getpwuid() .
$login = getlogin || (getpwuid($<))[0] ||
"jbloggs"; |
getpeername SOCKET |
Returns the packed sockaddr address of other end of the SOCKET connection.
$sockaddr = 'S n a4 x8'; #
An internet sockaddr
$hersockaddr = getpeername(S);
($family, $port, $heraddr) = unpack($sockaddr,$hersockaddr); |
getpgrp PID |
Returns the current process group for the specified PID (defaults to
current process), 0 for the current process. Produce a fatal error if used
on a system that doesn't support getpgrp. |
getppid |
Returns the process id of the parent process. |
getpriority WHICH,WHO |
Returns the current priority for a process, a process group, or a user.
Produces a fatal error if used on a system that doesn't support getpriority. |
getsockname SOCKET |
Returns the packed sockaddr address of this end of the SOCKET connection.
$sockaddr = 'S n a4 x8'; #
An internet sockaddr
$mysockaddr = getsockname(S);
($family, $port, $myaddr) = unpack($sockaddr,$mysockaddr); |
getsockopt SOCKET,LEVEL,OPTNAME |
Returns the socket option requested, or undefined if there is an error. |
glob EXPR |
Returns the value of EXPR with filename expansions such as a shell
would do. This is the internal function implementing the<*.*> operator. |
gmtime EXPR |
Converts a time (defaults to time) as returned
by the time function to a 9-element numeric array with the time analyzed
for the Greenwich timezone.
($sec,$min,$hour,$mday,$mon,$year,$wday,$yday,$isdst)
= gmtime($tNow);
$mon has the range 0..11, $wday has the range 0..6 and the year 2000
is returned as '100'. |
goto LABEL
goto &NAME |
The goto-LABEL form finds the LABEL statement and resumes execution
there. It may not be used where the target requires initialization, such
as a subroutine or a foreach loop. The goto-&NAME form substitutes
a call to the named subroutine for the currently running subroutine. This
is used by AUTOLOAD subroutines that wish to load another subroutine and
then pretend that the other subroutine had been called in the first place
(except that any modifications to @_
in the current subroutine are propagated to the other subroutine.) After
the goto, not even caller will be able to tell that
this routine was called first. |
grep BLOCK LIST
grep EXPR,LIST |
Evaluates the BLOCK or EXPR for each element of LIST, setting $_
to each element, returning the list value consisting of those elements
for which the expression evaluated to TRUE. In a scalar context, returns
the number of times the expression was TRUE.
@abc = grep(!/^#/, @xyz);
# weed out comments
or equivalently,
@abc = grep {!/^#/} @xyz;
# weed out comments
Since $_ is a reference into the list value, it can be used to modify
the elements of the array. |
hex EXPR |
Returns the decimal value of EXPR (defaults to $_)
interpreted as an hex string. See also oct. |
import |
There is no built-in import function. It is merely an ordinary
method subroutine defined (or inherited) by modules that wish to export
names to another module. The use function calls the
import
method for the package used. See also use. |
index STR,SUBSTR,POSITION
index STR,SUBSTR |
Returns the position of the first occurrence of SUBSTR in STR at or
after POSITION (defaults to start of string). The return value is based
at 0, or whatever the $[ variable
is set to. If the substring is not found, returns one less than the base,
commonly -1. |
int EXPR |
Returns the integer portion of EXPR (defaults to $_). |
join DELIM, LIST |
Combines the elements of the list, separating each with the specified
delimiter and returns the result. See also split.
$sCombined = join(':',$a,$b,$c); |
keys ASSOC_ARRAY |
Returns an array consisting of all the keys of the named associative
array, or the number of keys in a scalar context. The sequence is apparently
random, but consistent with that returned by values
and each.
@lKeys = keys(%ENV); |
kill LIST |
Sends a signal to a list of processes. The first element of the list
must be the signal to send. Returns the number of processes successfully
signaled. |
|
last LABEL |
The last command is used in loops (eg. while ...) and exits the loop
identified by the LABEL (current loop if omitted). The continue block,
if any, is not executed:
line: while (<STDIN>)
{
last line if /^$/;
# exit when done with header
...
} |
lc EXPR |
Returns the value of EXPR converted to lowercase. \L
in double-quoted strings. |
lcfirst EXPR |
Returns the value of EXPR with the first character conveted to lowercase.
\l
in double-quoted strings. |
length EXPR |
Returns the length in characters of the value of EXPR (defaults to
$_). |
link OLDFILE,NEWFILE |
Creates a new filename linked to the old filename. Returns 1 for success,
0 otherwise. |
listen SOCKET,QUEUESIZE |
Emulates the listen system call, returning True on success,
otherwise False. |
local EXPR |
Modifies the listed variables to be local to the enclosing block. If
more than one value is listed, the list must be placed in brackets. Local
works by saving the current values of those variables on a hidden stack
and restoring them upon exiting the block. Subroutines that are called
can reference the value of the local variable, but not the global one.
Commonly used to identify the parameters to a subroutine:
local($sEmployeeId,$sNewGrade,$sNotes) = @_;
local $result = '';
local $i; |
localtime EXPR |
Converts the value (defaults to time) to a 9-element
numeric array with the time analyzed for the local timezone.
($sec,$min,$hour,$mday,$mon,$year,$wday,$yday,$isdst)
= localtime($tNow);
$mon has the range 0..11, $wday has the range 0..6 and $year = 100
for '2000'.
In a scalar context, prints out the ctime value:
$sTime = localtime; # e.g. "Thu Sep 23
11:37:08 1998" |
log EXPR |
Returns logarithm (base e) of EXPR (defaults to $_). |
lstat FILEHANDLE
lstat EXPR |
Does the same thing as stat, but stats a symbolic
link instead of the file the symbolic link points to. If symbolic links
aren't implemented on the system, a normal stat is
done. |
map BLOCK LIST
map EXPR,LIST |
Evaluates the BLOCK or EXPR for each element of LIST (locally setting
$_ to each element) and returns the results of each evaluation in a list.
Evaluates BLOCK or EXPR in a list context, so each element of LIST may
produce zero, one, or more elements in the returned value.
@chars = map(chr, @nums);
# Numbers to chars
%hash = map {&key($_), $_} @array;
# Populate hash |
mkdir FILENAME,MODE |
Creates the directory specified by FILENAME, with permissions specified
by MODE. Returns True on success, otherwise False setting
$!. |
msgctl ID,CMD,ARG |
Calls the system function msgctl to retrieve the msqid_ds
structure. |
msgget KEY,FLAGS |
Calls thesSystem function msgget to retrieve the message queue
Id. |
msgsnd ID,MSG,FLAGS |
Calls the system function msgsnd to send the message to a queue. |
msgrcv ID,VAR,SIZE,TYPE,FLAGS |
Calls the system function msgrcv to receive a message from a
queue. |
my EXPR |
Like local, except with a greater level of privacy
- only the block within which the delration is made can 'see' the variable
- sub-routines do not. Built-in variables like $/
cannot be declared with my - use local instead. |
next LABEL |
The next command starts the next iteration of the loop:
Loop_1: while (<STDIN>)
# input read to $_
{
next Loop_1 if /^#/;
# discard comments
...
}
A continue block will get executed even on discarded lines. If the
LABEL is omitted, the command refers to the innermost enclosing loop. |
no Module LIST |
Opposite effect to use. |
oct EXPR |
Returns the decimal value of EXPR (defaults to $_)
interpreted as an octal string. If the value starts with '0x', it is interpreted
as a hex string instead. The following converts any decimal, octal, and
hex into its decimal equivalent:
$val = oct($val) if $val =~ /^0/; |
open FILEHANDLE,EXPR |
Opens the file whose filename is given by EXPR (defaults to scalar
variable with same name as the handle), and associates it with FILEHANDLE.
The handle may be an expression that returns the value to be used (eg.
a variable). A non-zero value is returned on success (PID of the subprocess
if the action involves a pipe), the undefined value otherwise. If the open
involved a pipe, the return value happens to be the pid of the subprocess. |
The first character of the filename dictates the mode (defaults
to read only): |
<
|
opened for input |
>
|
opened for output |
>>
|
opened for output by apending |
+
|
Opened for reading and writing (in front of the '<' or '>') |
|...
|
filename is interpreted as a command to which output is to be piped |
...|
|
filename is interpreted as a command from which output is to be piped |
-
|
on its own - opens StdIn |
>- |
on its own - opens StdOut |
Examples are: |
$INIFILE = '../config/process.ini';
open INIFILE or die "Unable to open $INIFILE
($!).\n"; |
open(LOGFILE, '>>./log_file');
open(ARTICLE, "caesar <$article |");
# decrypt article
open(extract, "|sort >/tmp/Tmp$$");
# $$ is the process id |
Alternatively, an EXPR beginning with ">&" can be specified, in
which case the rest of the string is interpreted as the name of a filehandle
(or file descriptor, if numeric) which is to be duped and opened. You may
use & after >,>>,<, +>, >> and <. The mode you specify should
match the mode of the original filehandle.
open(SAVEOUT, ">&STDOUT"); |
If you specify <&=N", where N is a number, then Perl will do
an equivalent of C's fdopen() of that file descriptor. For example:
open(FILEHANDLE, "<&=$fd") |
If you open a pipe on the command "-", i.e. either "|-" or "-|", then
there is an implicit fork done, and the return value of open is the pid
of the child within the parent process, and 0 within the child process.
Use defined($pid) to determine whether the open
was successful. The filehandle behaves normally for the parent, but i/o
to that filehandle is piped from/to the STDOUT/STDIN of the child process.
In the child process the filehandle isn't opened--i/o happens from/to the
new STDOUT or STDIN. Typically this is used like the normal piped open
when you want to exercise more control over just how the pipe command gets
executed, such as when you are running setuid, and don't want to have to
scan shell commands for metacharacters. The following pairs are more or
less equivalent:
open(PIPE1A, "|tr '[a-z]' '[A-Z]'");
open(PIPE1B, "|-") || exec 'tr', '[a-z]', '[A-Z]';
open(PIPE2A, "cat -n '$file'|");
open(PIPE2B, "-|") || exec 'cat', '-n', $file; |
Explicitly closing any piped filehandle causes the parent process to
wait for the child to finish, and returns the status value in $?.
On any operation which may do a fork, unflushed buffers remain unflushed
in both processes, which means you may need to set $|
to avoid duplicate output. |
opendir DIRHANDLE,EXPR |
Opens a directory named EXPR for processing by readdir()
, telldir() , seekdir() ,
rewinddir()
and closedir() . Returns True on success.
DIRHANDLEs have their own namespace separate from FILEHANDLEs. |
ord EXPR |
Returns the numeric ascii value of the first character of EXPR (defaults
to $_). |
pack TEMPLATE,LIST |
Takes an array or list of values and packs it into a binary structure,
returning the string containing the structure. The TEMPLATE is a sequence
of characters that give the order and type of values, as follows: |
A |
ASCII string (space padded) |
a |
ASCII string (null padded) |
b |
Bit string (ascending bit order) |
B |
Bit string (descending bit order) |
h |
Hex string (low nybble first) |
H |
Hex string (high nybble first) |
c |
Signed char |
C |
Unsigned char |
s |
Signed short |
S |
Unsigned short |
i |
Signed integer |
I |
Unsigned integer |
l |
Signed long |
L |
Unsigned long |
n |
Short in 'Network' order |
N |
Long in 'Network' order |
v |
Short in 'VAX' (little-endian) order |
V |
Long in 'VAX' (little-endian) order |
f |
Single-precision float |
d |
Double-precision float |
p |
Pointer to a null-terminated string. |
P |
pointer to a fixed-length string |
u |
UUencoded string |
x |
Null byte |
X |
Back up byte |
@ |
Null fill to absolute position |
Each letter may optionally be followed by a number which gives a repeat
count. With all types except "a", "A", "b", "B", "h" and "H", and "P" the
pack function will gobble up that many values from the LIST. A * for the
repeat count means to use however many items are left. The "a" and "A"
types gobble just one value, but pack it as a string of length count, padding
with nulls or spaces as necessary. |
pipe READHANDLE,WRITEHANDLE |
Opens a pair of connected pipes like the corresponding system call.
Note that Perl's pipes use StdIo buffering, so $|
may need to be set to flush the WRITEHANDLE after each command, depending
on the application. |
pop ARRAY |
Pops and returns the last value of the array, shortening the array
by 1. Has a similar effect to:
$tmp = $ARRAY[$#ARRAY--];
If there are no elements in the array, returns the undefined value. |
pos SCALAR |
Returns the offset returned by the most recent pattern match (m//g)
on the variable specified. May be modified to change that offset. |
print FILEHANDLE LIST |
Outputs the string data (defaults to $_)
to the specified Filehandle (defaults to StdOut, or the last selected
output channel).
print ("<html><head><title>$title</title></head><body>"); |
printf FILEHANDLE LIST |
Equivalent to a "print FILEHANDLE sprintf(LIST)". The first argument
of the list will be interpreted as the printf format. |
push ARRAY,LIST |
Treats ARRAY as a stack, and pushes the values of LIST onto the end
of ARRAY. The length of ARRAY increases by the length of LIST. Has the
same effect as:
for $value (LIST)
{ $ARRAY[++$#ARRAY] = $value; }
but is more efficient. Returns the new number of elements in the array. |
quotemeta EXPR |
Returns the value of EXPR with with all regular expression metacharacters
backslashed. This is the internal function implementing the \Q escape in
double-quoted strings. |
rand EXPR |
Returns a random fractional number between 0 and the value of EXPR
(should be positive, defaults to 1). This function produces repeatable
sequences unless srand is invoked. |
read FILEHANDLE,SCALAR,LENGTH,OFFSET |
Attempts to read LENGTH bytes of data into variable SCALAR from the
specified FILEHANDLE. Returns the number of bytes actually read, or undef
if there was an error. SCALAR will be grown or shrunk to the length actually
read. An OFFSET may be specified to place the read data at some other place
than the beginning of the string. This call is actually implemented in
terms of stdio's fread call. To get a true read system call, see sysread. |
readdir DIRHANDLE |
Returns the next directory entry for a directory opened by opendir.
If used in a list context, returns all the rest of the entries in the directory.
If there are no more entries, returns an undefined value in a scalar context
or a null list in a list context. |
readlink EXPR |
Returns the value of a symbolic link, if symbolic links are implemented.
If not, gives a fatal error. If there is some system error, returns the
undefined value and sets $! (errno).
EXPR defaults to $_ |
recv SOCKET,SCALAR,LEN,FLAGS |
Receives a message on a socket. Takes the same flags as the system
call of the same name. |
redo LABEL |
The redo command restarts the block within a loop without evaluating
the condition or executing the continue block. If the LABEL is omitted,
the command refers to the innermost enclosing loop.
Loop_01: while ($bRepeat)
{
...
redo Loop_01;
...
} |
ref EXPR |
Returns True if EXPR is a reference, otherwise False.
The value returned depends on the type of thing the reference is a reference
to. Builtin types include:
REF, SCALAR, ARRAY, HASH, CODE, GLOB |
Ref can be used as a typeof operator:
if (ref($r) eq "HASH")
{ print "r is a reference to an associative
array.\n"; }
if (!ref ($r)
{ print "r is not a reference.\n"; } |
rename OLDNAME,NEWNAME |
Changes the name of a file, returning True on success, otherwise
False. |
require EXPR |
Demands some semantics specified by EXPR (defaults to $_).
If EXPR is numeric, demands that the current version of Perl ($] or $PERL_VERSION
) be equal or greater than EXPR. Otherwise, demands that a library file
be included if it hasn't already been included (searching in @INC).
If EXPR is a bare word, assumes a " .pm " extension, following the approach
for standard modules. |
See also use. |
reset EXPR |
Generally used in a continue block at the end of a loop to clear variables
and reset ?? searches so that they work again. The expression is interpreted
as a list of single characters (hyphens allowed for ranges). All variables
and arrays beginning with one of those letters are reset to their pristine
state. If the expression is omitted, one-match searches (?pattern?) are
reset to match again. Only resets variables or searches in the current
package. Always returns 1. Examples:
reset 'X';
# reset all X variables
reset 'a-z';
# reset lower case variables
reset;
# just reset ?? searches |
return LIST |
Returns from a subroutine or eval with the value specified. In the
absence of a return a subroutine or eval will automatically return the
value of the last expression evaluated. |
reverse LIST |
In a list context, returns the elements of LIST in the opposite order.
In a scalar context, returns the first element of LIST in the opposite
order. |
rewinddir DIRHANDLE |
Sets the current position to the beginning of the directory for the
readdir
routine on DIRHANDLE. |
rindex STR,SUBSTR,POSITION |
Works just like index except that it returns the
position of the LAST occurrence of SUBSTR in STR. If POSITION is specified,
returns the last occurrence at or before that position. |
rmdir FILENAME |
Deletes the directory specified by FILENAME (defaults to $_)
if it is empty, returning True on success, otherwise False
and sets $! (errno). |
scalar EXPR |
Forces EXPR to be interpreted in a scalar context and returns the value
of EXPR. |
seek FILEHANDLE,POSITION,WHENCE |
Randomly positions the file pointer for FILEHANDLE, returning 1 on
success, otherwise 0. The values for WHENCE are: |
0 |
SEEK_SET |
set the file pointer to POSITION |
1 |
SEEK_CUR |
set the file pointer to current plus POSITION |
2 |
SEEK_END |
set the file pointer to EOF plus offset |
seekdir DIRHANDLE,POS |
Sets the current position for the readdir routine
on DIRHANDLE. POS must be a value returned by telldir. |
select FILEHANDLE |
Sets the current default filehandle for output (initially STDOUT) if
FILEHANDLE is supplied and returns the currently selected filehandle. A
write
or a print without a filehandle will default to this
value, and references to variables related to output will refer to this
output channel. For example, if you have to set the top of form format
for more than one output channel, you might do the following:
select(REPORT1);
$^ = 'report1_top';
select(REPORT2);
$^ = 'report2_top';
FILEHANDLE may be an expression whose value gives the name of the actual
filehandle.
$oldfh = select(STDERR); $| = 1; select($oldfh);
With Perl 5, filehandles are objects with methods, and the last example
is preferably written:
use FileHandle;
STDERR->autoflush(1); |
select RBITS,WBITS,EBITS,TIMEOUT |
Calls the select system call with the bitmasks and timeout specified,
which can be constructed using fileno and vec,
along these lines:
$rin = $win = $ein = '';
vec($rin,fileno(STDIN),1) = 1;
vec($win,fileno(STDOUT),1) = 1;
$ein = $rin | $win;
($nfound,$timeleft) =
select($rout=$rin, $wout=$win, $eout=$ein,
$timeout);
or to block until something becomes ready:
$nfound = select($rout=$rin, $wout=$win, $eout=$ein,
undef);
A 250 microsecond pause can be accomplished by:
select(undef, undef, undef, 0.25); |
semctl ID,SEMNUM,CMD,ARG |
Calls the system function semctl to access the semaphore value
array. |
semget KEY,NSEMS,FLAGS |
Calls the system function semget to retrieve the semaphore id. |
semop KEY,OPSTRING |
Calls the system function semop to perform semaphore operations
such as signaling and waiting. |
send SOCKET,MSG,FLAGS,TO |
Sends a message on a socket returning the number of characters sent.
Takes the same flags as the system call of the same name. |
setpgrp PID,PGRP |
Sets the current process group for the specified PID, 0 for the current
process. |
setpriority WHICH,WHO,PRIORITY |
Sets the current priority for a process, a process group, or a user. |
setsockopt SOCKET,LEVEL,OPTNAME,OPTVAL |
Sets the socket option requested, returning undefined if there is an
error. |
shift ARRAY |
Removes the first entry in an Array (position '0') and moves all other
elemnts to the left. Returns the value removed.
$value = shift (@values); |
shmctl ID,CMD,ARG |
Calls the system function shmctl to retrieve the shmid_ds structure. |
shmget KEY,SIZE,FLAGS |
Calls the system function shmget to retrieve the shared memory
segment id. |
shmread ID,VAR,POS,SIZE
shmwrite ID,STRING,POS,SIZE |
Reads or writes the System shared memory segment ID starting at position
POS for size SIZE by attaching to it, copying in/out, and detaching from
it, returning True on success. When reading, VAR will hold the data
read, and when writing, if STRING is too short, nulls are written to fill
out to SIZE bytes. |
shutdown SOCKET,HOW |
Shuts down a socket connection. |
sin EXPR |
Returns the sine of EXPR, expressed in radians (defaults to $_). |
sleep EXPR |
Causes the script to sleep for EXPR seconds, or forever if no EXPR.
May be interrupted by sending the process a SIGALRM. Returns the number
of seconds actually slept. |
socket SOCKET,DOMAIN,TYPE,PROTOCOL |
Opens a socket of the specified kind and attaches it to filehandle
SOCKET. |
socketpair SOCKET1,SOCKET2,DOMAIN,TYPE,PROTOCOL |
Creates an unnamed pair of sockets in the specified domain, of the
specified type. |
sort SUBNAME LIST
sort BLOCK LIST
sort LIST |
Sorts the LIST and returns the result, ignoring undefined values. If
SUBNAME (optionally named by a scalar) or BLOCK are specified, the corresponding
subroutine or code returns a value that is less than, equal to, or greater
than 0, depending on how the elements of the array are to be ordered. In
place of a SUBNAME, you can provide a BLOCK as an anonymous, in-line sort
subroutine. The default is to sort in standard string comparison order. |
The normal calling code for subroutines is bypassed, such that it may
not be recursive, and the two elements to be compared are passed as references
$a and $b. |
@sorted = sort @raw;
@sorted = sort {$b cmp $a} @raw; # backwards
sub ascending { $a <=> $b; }
@sorted = sort ascending $raw; # Numeric
order |
splice ARRAY,OFFSET,LENGTH,LIST |
Removes the elements designated by OFFSET and LENGTH (defaults to rest
of array) from an array, and replaces them with the elements of LIST (if
specified), returning the elements removed from the array. |
split /PATTERN/,EXPR,LIMIT |
Splits the EXPR (defaults to $_)
into an array of strings using the pattern as delimiter (defaults to whitespace
/[ \t\n]+/) and returns the results. In a scalar context, returns
the number of fields found and results can be split into the @_
array if ?? are used as the pattern delimiters. |
If LIMIT is specified and is not negative, splits into no more than
that many fields. If it is not specified, trailing null fields are
ignored. |
If the PATTERN contains parentheses, additional array elements are
created from each matching substring in the delimiter:
split(/([-\])/, "1\Jan-2000");
produces the list value:
(1,'\',Jan,'-',2000) |
sprintf FORMAT,LIST |
Returns a string formatted by the printf conventions of C. |
sqrt EXPR |
Return the square root of EXPR (defaults to $_). |
srand EXPR |
Sets the random number seed for the rand operator
(defaults to srand(time)). |
stat FILEHANDLE
stat EXPR |
Returns a 13-element array giving the status info for a file, either
previously opened via FILEHANDLE, or named by EXPR:
($dev,$ino,$mode,$nlink,$uid,$gid,$rdev,$size,
$atime,$mtime,$ctime,$blksize,$blocks)
=
stat($filename); |
If stat is passed just an underline (a special filehandle), the current
contents of the stat structure from the last stat or filetest are returned. |
study SCALAR |
Builds a linked list of every character in the variable (defaults to
$_)
in preparation for preforming many pattern matches on the string before
it is next modified. Whether this action is beneficial depends on the nature
and number of patterns to be used, and on the distribution of characters
in the string. Loops that scan for many short constant strings will benefit
most, and only one study active can be active at a time. |
substr EXPR,OFFSET,LEN |
Extracts a substring from EXPR starting at the specified OFFSET (first
character is at offset $[, defaults
to 0). If OFFSET is negative, starts that far from the end of the string.
If LEN is omitted, returns everything to the end of the string. |
symlink OLDFILE,NEWFILE |
Creates a new filename symbolically linked to the old filename, returning
1 on success. |
syscall LIST |
Calls the system call specified as the first element of the list, passing
the remaining elements as arguments to the system call. |
sysread FILEHANDLE,SCALAR,LENGTH,OFFSET |
Attempts to read a number of bytes of data into a variable from the
specified FILEHANDLE, using the system call read, returning the
number of bytes actually read. The OFFSET may be specified to place the
read data at some other place than the beginning of the string. |
system LIST |
Does exactly the same thing as "exec LIST" except
that a fork is done first, and the parent process waits for the child process
to complete. |
syswrite FILEHANDLE,SCALAR,LENGTH,OFFSET |
Attempts to write a number of bytes of data from a variable to the
specified FILEHANDLE, using the system call write, returning the
number of bytes actually written. An OFFSET may be specified to retrieve
the data from some other place than the beginning of the string. |
tell FILEHANDLE |
Returns the current file position for FILEHANDLE (defaults to the file
last read). |
telldir DIRHANDLE |
Returns the current position of the readdir
routines on DIRHANDLE. |
tie VARIABLE,PACKAGENAME,LIST |
This function binds a variable to a package that will provide the implementation
for the variable. VARIABLE is the name of the variable to be enchanted.
PACKAGENAME is the name of a package implementing objects of correct type.
Any additional arguments are passed to the "new" method of the package.
Typically these are arguments such as might be passed to the dbm_open
function of C.
# print out history file offsets
tie(%HIST, NDBM_File, '/usr/lib/news/history',
1, 0);
while (($key,$val) = each %HIST)
{ print $key, ' = ', unpack('L',$val),
"\n"; }
untie(%HIST); |
A package implementing the following types of data should have the
specified methods: |
Associative Array |
TIEHASH objectname, LIST
DESTROY this
FETCH this, key
STORE this, key, value
DELETE this, key
EXISTS this, key
FIRSTKEY this
NEXTKEY this, lastkey |
Ordinary Array |
TIEARRAY objectname, LIST
DESTROY this
FETCH this, key
STORE this, key, value
[others TBD] |
Scalar |
TIESCALAR objectname, LIST
DESTROY this
FETCH this,
STORE this, value |
time |
Returns the number of non-leap seconds since 00:00:00 UTC, January
1, 1970. |
times |
Returns a four-element array giving the user and system times, in seconds,
for this process and the children of this process.
($user,$system,$cuser,$csystem) = times; |
truncate FILEHANDLE,LENGTH |
Truncates the file opened on FILEHANDLE (or named by by a variable)
to the specified length. |
uc EXPR |
Returns the value of EXPR converted to uppercase. \U in double-quoted
strings. |
ucfirst EXPR |
Returns the value of EXPR with the first character conveted to uppercase.
\u in double-quoted strings. |
umask EXPR |
Sets the umask for the process (if specified) and returns the old one. |
undef EXPR |
Undefines the value of EXPR, which must be a scalar , an array, or
a subroutine name (using "&"). Returns an undefined value, even where
the expression is ommitted. |
unlink LIST |
Deletes a list of files, returning the number of files successfully
deleted.
$iCount = unlink <*.bak>;
Use rmdir to delete directories. |
unpack TEMPLATE,EXPR |
Performs the reverse of pack, expanding a string
into a list value, based on the TEMPLATE (same format as in the pack function). |
This example performs the equivalent to substring:
sub substr
{
local($what,$where,$howmuch)
= @_;
unpack("x$where a$howmuch",
$what);
} |
untie VARIABLE |
Breaks the binding between a variable and a package. See also tie. |
unshift ARRAY,LIST |
Prepends list to the front of the array, and returns the new number
of elements in the array. See also shift and push. |
use Module LIST |
Imports some semantics into the current package from the named module,
generally by aliasing certain subroutine or variable names into your package,
but alters the namespace unlike require. |
utime LIST |
Changes the access and modification times on each file of a list of
files. The first two elements of the list must be the NUMERICAL access
and modification times, in that order. Returns the number of files successfully
changed. |
values ASSOC_ARRAY |
Returns an array containing all of the values from the associative
array, or the number of values in a scalar context. The values are returned
in the same order as the keys or each
functions. |
vec EXPR,OFFSET,BITS |
Retrieve the value of the bitfield specified in the string treated
as a vector of unsigned integers. BITS must be a power of two from 1 to
32. Vectors created with vec() can also be manipulated with the logical
operators |, & and ^, which will assume a bit vector operation is desired
when both operands are strings. |
wait |
Waits for a child process to terminate, returning the pid of the deceased
process, or -1 if there are none. The status is returned in $?. |
waitpid PID,FLAGS |
Waits for the specified child process to terminate, returning the pid
of the deceased process, or -1 if there are none. The status is returned
in $?. |
wantarray |
Returns True if the context of the currently executing subroutine
is looking for a list value, otherwise False if the context is looking
for a scalar.
return wantarray # Check
context
? ()
# Return undefind array
: undef;
# Return undefined scalar |
warn LIST |
Produces a message on StdErr just like die,
but doesn't exit or throw an exception. |
write FILEHANDLE |
Writes a formatted record (possibly multi-line) to the specified file,
using the format associated with that file. By default the format for a
file is the one having the same name is the filehandle. |
Top of form processing is handled automatically: if there is insufficient
room on the current page for the formatted record, the page is advanced
by writing a form feed, a special top-of-page format is used to format
the new page header, and then the record is written. By default the top-of-page
format is the name of the filehandle with "_TOP" appended, but it may be
dynamically set to the format of your choice by assigning the name to the
$^ variable while the filehandle is selected. The number of lines remaining
on the current page is in variable $-, which can be set to 0 to force a
new page. |
This is not the opposite of read. |
|
|